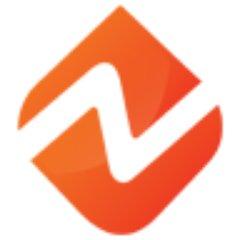
Install
Retry utility
If you find this useful, please consider supporting my work with a donation or nominate me for a GitHub Star.
Description
A utility for retrying failed async JavaScript calls based on the error returned.
Usage
Node.js
npm install @humanwhocodes/retry
# or
yarn add @humanwhocodes/retry
Import into your Node.js project:
// CommonJS
const { Retrier } = require("@humanwhocodes/retry");
// ESM
import { Retrier } from "@humanwhocodes/retry";
Deno
Install using JSR:
deno add @humanwhocodes/retry
#or
jsr add @humanwhocodes/retry
Then import into your Deno project:
import { Retrier } from "@humanwhocodes/retry";
Bun
Install using this command:
bun add @humanwhocodes/retry
Import into your Bun project:
import { Retrier } from "@humanwhocodes/retry";
Browser
It's recommended to import the minified version to save bandwidth:
import { Retrier } from "https://cdn.skypack.dev/@humanwhocodes/retry?min";
However, you can also import the unminified version for debugging purposes:
import { Retrier } from "https://cdn.skypack.dev/@humanwhocodes/retry";
API
After importing, create a new instance of Retrier
and specify the function to run on the error. This function should return true
if you want the call retried and false
if not.
// this instance will retry if the specific error code is found
const retrier = new Retrier(error => {
return error.code === "ENFILE" || error.code === "EMFILE";
});
Then, call the retry()
method around the function you'd like to retry, such as:
import fs from "fs/promises";
const retrier = new Retrier(error => {
return error.code === "ENFILE" || error.code === "EMFILE";
});
const text = await retrier.retry(() => fs.readFile("README.md", "utf8"));
The retry()
method will either pass through the result on success or wait and retry on failure. Any error that isn't caught by the retrier is automatically rejected so the end result is a transparent passing through of both success and failure.
Developer Setup
- Fork the repository
- Clone your fork
- Run
npm install
to setup dependencies - Run
npm test
to run tests
License
Apache 2.0
Prior Art
This utility is inspired by, and contains code from graceful-fs
.