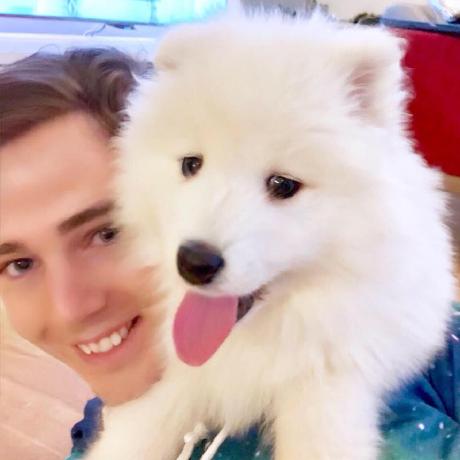
Install
load-grunt-tasks 
Load multiple grunt tasks using globbing patterns
Usually you would have to load each task one by one, which is unnecessarily cumbersome.
This module will read the dependencies
/devDependencies
/peerDependencies
/optionalDependencies
in your package.json and load grunt tasks that match the provided patterns.
Before
grunt.loadNpmTasks('grunt-shell');
grunt.loadNpmTasks('grunt-sass');
grunt.loadNpmTasks('grunt-recess');
grunt.loadNpmTasks('grunt-sizediff');
grunt.loadNpmTasks('grunt-svgmin');
grunt.loadNpmTasks('grunt-styl');
grunt.loadNpmTasks('grunt-php');
grunt.loadNpmTasks('grunt-eslint');
grunt.loadNpmTasks('grunt-concurrent');
grunt.loadNpmTasks('grunt-bower-requirejs');
After
require('load-grunt-tasks')(grunt);
Install
$ npm install --save-dev load-grunt-tasks
Usage
// Gruntfile.js
module.exports = grunt => {
// Load all grunt tasks matching the ['grunt-*', '@*/grunt-*'] patterns
require('load-grunt-tasks')(grunt);
grunt.initConfig({});
grunt.registerTask('default', []);
};
Examples
Load all grunt tasks
require('load-grunt-tasks')(grunt);
Equivalent to:
require('load-grunt-tasks')(grunt, {pattern: ['grunt-*', '@*/grunt-*']});
Load all grunt-contrib tasks
require('load-grunt-tasks')(grunt, {pattern: 'grunt-contrib-*'});
Load all grunt-contrib tasks and another non-contrib task
require('load-grunt-tasks')(grunt, {pattern: ['grunt-contrib-*', 'grunt-shell']});
Load all grunt-contrib tasks excluding one
You can exclude tasks using the negate !
globbing pattern:
require('load-grunt-tasks')(grunt, {pattern: ['grunt-contrib-*', '!grunt-contrib-coffee']});
Set custom path to package.json
require('load-grunt-tasks')(grunt, {config: '../package'});
Only load from devDependencies
require('load-grunt-tasks')(grunt, {scope: 'devDependencies'});
Only load from devDependencies
and dependencies
require('load-grunt-tasks')(grunt, {scope: ['devDependencies', 'dependencies']});
All options in use
require('load-grunt-tasks')(grunt, {
pattern: 'grunt-contrib-*',
config: '../package.json',
scope: 'devDependencies',
requireResolution: true
});
Options
pattern
Type: string | string[]
Default: ['grunt-*', '@*/grunt-*']
(Glob pattern)
config
Type: string | object
Default: Path to nearest package.json
scope
Type: string | string[]
Default: ['dependencies', 'devDependencies', 'peerDependencies', 'optionalDependencies']
Values: 'dependencies'
, 'devDependencies'
, 'peerDependencies'
, 'optionalDependencies'
, 'bundledDependencies'
requireResolution
Type: boolean
Default: false
Traverse up the file hierarchy looking for dependencies like require()
, rather than the default grunt-like behavior of loading tasks only in the immediate node_modules
directory.