10 Tips for Implementing Auto Hide in Vue JS 3 Component Toasts
Introduction:
Tip 1: Understand the purpose of auto hide
Tip 2: Choose a suitable duration for auto hide
Tip 3: Customize auto hide behavior
Tip 4: Consider user interaction scenarios
Tip 5: Include visual cues during the hiding process
Tip 6: Test across various devices and screen sizes
Tip 7: Handle edge cases gracefully
Tip 8: Provide clear feedback after hiding toast
Tip 9: Optimize performance
Tip 10: Stay updated with Vue JS updates and best practices
Conclusion:
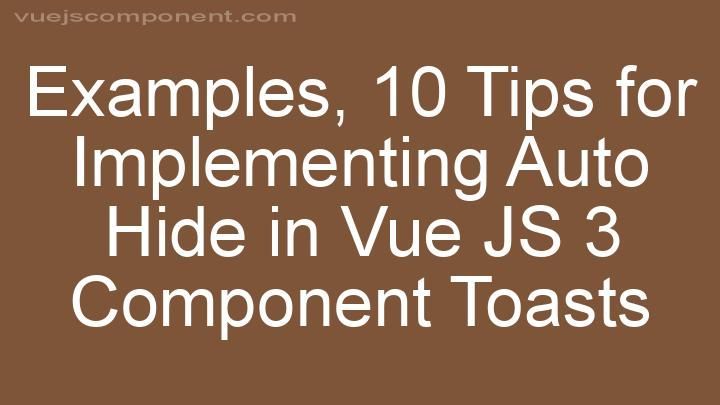
Introduction:
As developers, we strive to create user-friendly and interactive web applications. One way to enhance the user experience is by implementing auto hide functionality in Vue JS 3 component toasts. Auto hide allows the toast to disappear after a certain duration, reducing clutter and improving the flow of information. In this blog post, we will explore ten tips to help you effectively implement auto hide in your Vue JS 3 component toasts. So, let's get started!
Tip 1: Understand the purpose of auto hide
Auto hide functionality in component toasts serves the purpose of providing timely information to users without overwhelming them. By automatically hiding the toast after a specific duration, we ensure that users receive the necessary information without disrupting their workflow. It enhances the overall user experience and keeps the interface clean and organized.
Tip 2: Choose a suitable duration for auto hide
Selecting an appropriate time interval for auto hiding is crucial. If the duration is too short, users might not have enough time to read the toast. On the other hand, if the duration is too long, it might create an unnecessary delay in the user's workflow. Consider the complexity of the information being displayed and the reading speed of your target audience to determine the ideal duration.
Tip 3: Customize auto hide behavior
Vue JS 3 provides various options to customize the auto hide behavior according to your specific requirements. You can control when the auto hide starts, whether it pauses on hover or user interaction, and how it transitions when hiding. Take advantage of these options to create a seamless auto hide experience that aligns with your application's design and functionality.
Tip 4: Consider user interaction scenarios
While implementing auto hide, it's essential to consider different user interaction scenarios. For example, if a user hovers over the toast, the auto hide timer could pause to allow them to read the content. Additionally, if a user clicks on the toast, you might want to cancel the auto hide behavior entirely. By handling these scenarios gracefully, you provide a more intuitive and user-friendly experience.
Tip 5: Include visual cues during the hiding process
Visual cues play a significant role in guiding users through the auto hide process. Adding animation or transition effects to the toast as it disappears can provide a smooth and visually appealing experience. Consider using fade-out animations or slide-up transitions to indicate that the toast is about to disappear. These visual cues help users understand what's happening and prevent any confusion.
Tip 6: Test across various devices and screen sizes
To ensure a seamless auto hide functionality, it's crucial to test your implementation across different devices and screen sizes. Viewport dimensions can vary significantly, and what works perfectly on one device may not translate well to another. By testing on various devices, you can identify any issues that may arise due to different screen sizes and ensure a consistent experience for all users.
Tip 7: Handle edge cases gracefully
When implementing auto hide, it's essential to consider potential edge cases. For example, what happens when multiple toasts appear simultaneously? How do you handle overlapping messages without causing confusion? By implementing proper error handling techniques and considering complex scenarios, you can ensure that your auto hide functionality remains smooth and error-free.
Tip 8: Provide clear feedback after hiding toast
After a toast hides, it's essential to provide clear feedback to the user. Consider displaying a success message or an icon to indicate completion. This feedback reassures the user that their action was successful and helps maintain a positive user experience. By providing clear feedback, you ensure that users are aware that the toast has disappeared and can proceed with their tasks.
Tip 9: Optimize performance
Optimizing the performance of your auto hide functionality is crucial for a smooth user experience. Techniques such as debouncing or throttling can prevent excessive triggers and ensure that the auto hide behavior is triggered only when necessary. Additionally, Vue JS 3 provides built-in features like v-show
and v-if
that can help optimize rendering performance. By implementing these techniques, you can create a responsive and efficient auto hide feature.
Tip 10: Stay updated with Vue JS updates and best practices
Vue JS is a rapidly evolving framework, and staying up-to-date with the latest updates and best practices is essential. The Vue JS community continually introduces new features and improvements that can enhance your auto hide implementation. By staying informed, you can take advantage of these updates to improve the functionality and user experience of your Vue JS 3 component toasts.
Conclusion:
Implementing auto hide functionality in Vue JS 3 component toasts can significantly enhance the user experience of your web application. By following these ten tips, you can ensure that your auto hide feature is seamless, user-friendly, and visually appealing. Remember to consider the purpose of auto hide, choose suitable durations, customize the behavior, and handle edge cases gracefully. Additionally, provide clear feedback to the user, optimize performance, and stay updated with Vue JS updates and best practices. We hope these tips help you create outstanding auto hide functionality in your Vue JS 3 projects. Happy coding!
FREQUENTLY ASKED QUESTIONS
How can I implement auto hide functionality in Vue JS 3 component toasts?
To implement auto hide functionality in Vue JS 3 component toasts, you can use a combination of Vue's lifecycle hooks and a timer. Here's a step-by-step guide:
-
First, define a data property in your toast component to keep track of whether the toast should be visible or hidden. Let's call it
showToast
and initialize it asfalse
. -
In the
mounted
lifecycle hook of your component, start a timer usingsetTimeout
. Set the duration of the timer to the desired time in milliseconds, after which you want the toast to automatically hide. -
Inside the
setTimeout
callback function, update theshowToast
property tofalse
, indicating that the toast should be hidden. -
In the template of your component, use
v-if
orv-show
directive to conditionally render the toast based on the value ofshowToast
. This will ensure that the toast is displayed whenshowToast
istrue
and hidden when it'sfalse
.
Here's an example code snippet to illustrate the implementation:
<template>
<div>
<div v-show="showToast" class="toast">
<!-- Toast content goes here -->
</div>
</div>
</template>
<script>
export default {
data() {
return {
showToast: false
}
},
mounted() {
setTimeout(() => {
this.showToast = false;
}, 3000); // Auto hide after 3 seconds (adjust the duration as per your requirement)
}
}
</script>
<style>
.toast {
/* Styles for the toast */
}
</style>
In this example, the toast will be displayed initially (showToast
is set to true
). After 3 seconds (setTimeout
duration), the showToast
property will be updated to false
, hiding the toast automatically.
Feel free to adjust the code as per your specific requirements, such as adding animations or customizing the toast appearance. Happy coding!
Can you provide an example of implementing auto hide in Vue JS 3 component toasts?
Certainly! Here's an example of how you can implement auto hide in Vue JS 3 component toasts:```vue
In this example, we have a button that triggers the display of a toast message. The `showToast` method sets the necessary data properties to show the toast and assigns the desired type and text to be displayed.
To implement the auto hide functionality, we use the `setTimeout` function to set a timer of 3 seconds. After 3 seconds, the `toastVisible` property is set to false, which hides the toast.
You can customize the toast appearance by modifying the CSS classes in the `<style>` section. In this example, we have defined two classes: `success` and `error`, which define the background color for different types of toasts.
Feel free to adjust the code according to your specific requirements. Hope this helps!
Are there any additional tips for implementing auto hide in Vue JS 3 component toasts?
Certainly! When implementing auto hide in Vue JS 3 component toasts, there are a few additional tips that can help you achieve the desired functionality:
-
Utilize the v-show directive: Vue provides the v-show directive, which allows you to conditionally show or hide an element based on a given expression. You can bind this directive to a boolean data property that controls the visibility of your toast component.
-
Set a timer for auto hide: To make the toast automatically hide after a certain duration, you can use JavaScript's setTimeout function. Inside the mounted lifecycle hook of your toast component, you can call setTimeout and set the duration for how long the toast should be visible before hiding itself.
-
Update the visibility property: To ensure that the toast is hidden after the specified duration, you need to update the visibility property that you bound to the v-show directive. You can accomplish this by using the Vue's data object and modifying the boolean value to false inside the setTimeout callback function.
Here's an example implementation:
<template>
<div v-show="isVisible" class="toast">
<!-- Your toast content here -->
</div>
</template>
<script>
export default {
data() {
return {
isVisible: true,
};
},
mounted() {
setTimeout(() => {
this.isVisible = false;
}, 3000); // Hide the toast after 3 seconds
},
};
</script>
<style>
.toast {
/* Your toast styles here */
}
</style>
With these additional tips, you should be able to implement auto hide functionality in your Vue JS 3 component toasts. Feel free to customize the duration and styles according to your needs. If you have any further questions, feel free to ask!
What is auto hide in Vue JS 3 component toasts?
In Vue JS 3 component toasts, "auto hide" refers to the feature that automatically hides the toast message after a certain duration. When enabled, the toast message will be displayed for a specified period of time and then disappear without any user interaction. This is useful for displaying temporary notifications or alerts to the user without requiring them to manually dismiss the message. The duration of the auto hide can be configured based on your preferences and the specific requirements of your application.