Mastering the Art of Dynamic Styling: Adding Computed Properties to CSS with v-bind in Vue 3
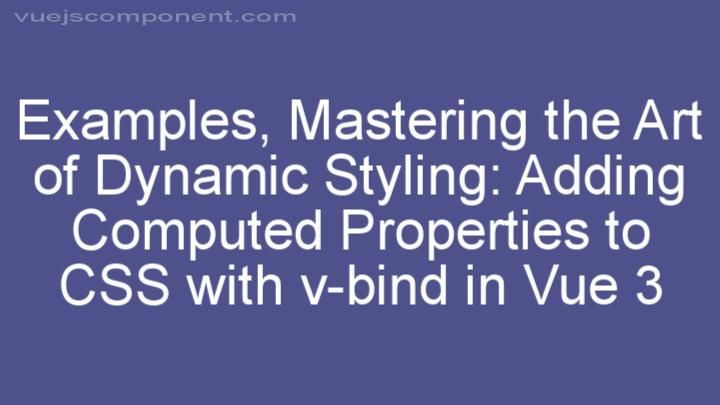
Introduction:
Welcome, fellow developers, to the exciting world of dynamic styling in Vue 3! Today, we're going to dive into the powerful feature of computed properties and how they can revolutionize your CSS game. With the help of v-bind, we'll unlock a whole new level of responsiveness and flexibility in our Vue projects. So buckle up and get ready to take your styling skills to the next level!
I. Understanding Computed Properties in Vue 3:
First things first, let's talk about computed properties and their role in Vue 3. In simple terms, computed properties are special properties that are calculated based on other properties in your Vue instance. They are like magic formulas that allow you to transform and manipulate your data in real-time. Unlike regular data properties or methods, computed properties are cached and only update when their dependencies change. This makes them perfect for dynamic styling scenarios.
So why should you bother with computed properties instead of just using regular properties or methods? Well, computed properties offer some unique advantages. First, they provide a clean and concise syntax for performing complex calculations or transformations on your data. Instead of cluttering your template with complex logic, you can encapsulate it neatly within a computed property. Second, computed properties automatically update whenever the data they depend on changes. This means that your styles will always stay in sync with your data, without any extra effort on your part.
II. Getting Started with v-bind:
Now that we understand the power of computed properties, let's see how v-bind can take our dynamic styling to the next level. In Vue 3, v-bind is a directive that allows us to bind the value of an HTML attribute or CSS property to a computed property. This means that we can dynamically apply styles based on specific conditions or calculations.
To use v-bind, simply add a colon before the attribute or property you want to bind. For example, let's say we have a computed property called "backgroundColor" that calculates the background color based on some data. We can bind this property to the "style" attribute of an element like this:
Now, whenever the value of "backgroundColor" changes, the style of the element will automatically update. Pretty neat, right?
III. Exploring Advanced Usage:
While v-bind is great for basic dynamic styling, it can also be combined with conditional statements (v-if) for more advanced scenarios. Let's say we want to display a different background color based on a certain condition. We can use v-bind together with v-if to achieve this:
In this example, the background color will change dynamically based on the value of the "isDarkMode" property. This allows us to create dynamic themes or responsive layouts with ease.
But v-bind doesn't stop at binding just one CSS property. We can use it to bind multiple properties at once by using an object syntax. For example:
This way, we can create a fully dynamic and responsive component by binding multiple CSS properties to our computed properties.
To optimize performance when using computed properties and v-bind, keep in mind that the computed properties will be recalculated whenever any of their dependencies change. If you have a large number of dependencies or complex calculations, this could impact performance. In such cases, consider using a combination of data properties and methods instead of computed properties.
IV. Real-world Examples:
Now that we have a solid understanding of computed properties and v-bind, let's explore some real-world examples where they can be applied creatively. One practical use case is creating responsive layouts. By binding the width, height, and positioning properties of an element to computed properties that calculate the desired dimensions based on the viewport size, we can achieve a responsive design that adapts to different devices.
Another exciting application is theme switching. By binding various CSS properties to computed properties that determine the color palette, font styles, and other theme-related attributes, we can easily switch between different themes with just a few lines of code. This allows us to provide a customizable and personalized experience to our users.
Let's take a look at a code snippet for theme switching:
In this example, the background color, text color, and font family will change dynamically based on the value of the "isDarkMode" property. This demonstrates the flexibility and power of computed properties and v-bind in creating dynamic and customizable user experiences.
V. Troubleshooting Common Issues:
As with any technology, using computed properties and v-bind may come with its fair share of challenges. One common issue is not updating the dependencies correctly. Make sure that all the properties your computed property relies on are correctly declared as dependencies. If a dependency is missing, your computed property may not update as expected.
Another issue to watch out for is inadvertently creating circular dependencies. If two computed properties depend on each other, it can lead to an infinite loop of recalculations. To avoid this, carefully analyze the dependencies of your computed properties and ensure they form a clear and non-circular chain.
If you encounter any issues, a helpful tip for debugging is to console.log the computed properties and their dependencies. This can give you valuable insights into the flow of data and help you identify any issues in your logic.
Conclusion:
Congratulations on mastering the art of dynamic styling with computed properties and v-bind in Vue 3! We've explored the power and versatility of computed properties, learned how to use v-bind to dynamically apply styles, and even dabbled in more advanced scenarios. By creatively applying these techniques, you can create stunning and responsive designs that truly elevate your Vue projects.
Remember, practice makes perfect. So don't be afraid to experiment and explore the possibilities. And if you ever run into any challenges, refer back to this guide for troubleshooting tips and solutions. With your newfound knowledge, there's no limit to what you can achieve with dynamic styling in Vue 3.
Now go forth, fellow developers, and unleash the full potential of computed properties and v-bind in your CSS wizardry! Happy coding!
FREQUENTLY ASKED QUESTIONS
What are computed properties in Vue 3?
Computed properties in Vue 3 are a powerful feature that allow you to dynamically calculate and update values based on the data in your Vue instance. They are like functions that automatically update whenever their dependencies change.In Vue 3, computed properties are defined using the computed
option in the component's options object. You can define a computed property as a getter function, which returns the computed value based on some data in your component.
Here's an example to illustrate how computed properties work in Vue 3:
export default {
data() {
return {
firstName: 'John',
lastName: 'Doe'
}
},
computed: {
fullName() {
return this.firstName + ' ' + this.lastName;
}
}
}
In this example, we have a fullName
computed property that concatenates the firstName
and lastName
data properties together. Whenever either the firstName
or lastName
changes, the fullName
computed property will automatically update to reflect the new value.
Computed properties are especially useful when you have complex logic or calculations that depend on multiple data properties. By using computed properties, you can keep your template code clean and concise, as the computed property will handle the calculations for you.
You can access computed properties in your template just like any other data property. For example, in your template, you can use {{ fullName }}
to display the computed value.
Overall, computed properties in Vue 3 offer a convenient way to calculate and update values based on the data in your component, making your code more concise and maintainable.
How can I add computed properties to CSS using v-bind in Vue 3?
To add computed properties to CSS using v-bind in Vue 3, you can follow these steps:
-
First, create a computed property in your Vue component. Computed properties are dynamic properties that are calculated based on other data properties.
-
Inside the computed property, define the CSS properties and their values using JavaScript. You can use template literals to concatenate dynamic values if needed.
-
In your template, use the v-bind directive to bind the computed property to the style attribute of the element you want to apply the CSS to.
Here's an example:
<template>
<div :style="computedStyles"></div>
</template>
<script>
export default {
computed: {
computedStyles() {
return {
backgroundColor: 'red',
color: 'white',
fontSize: `${this.fontSize}px`
}
},
fontSize() {
// Your logic to calculate the font size dynamically
return 16;
}
}
}
</script>
In the example above, the computedStyles property is an object that contains CSS properties and their values. The backgroundColor, color, and fontSize properties are dynamic and can be changed based on your application's logic. The fontSize property is another computed property that calculates the font size dynamically.
By using v-bind, we bind the computedStyles object to the style attribute of the <div>
element, which applies the computed CSS styles to the element.
Remember to replace the values and logic in the example with your own requirements. This approach allows you to create dynamic CSS properties based on the data in your Vue component.
Can you give an example of adding computed properties to CSS with v-bind in Vue 3?
Certainly! In Vue 3, you can add computed properties to CSS using the v-bind directive. Here's an example to illustrate how it works:Let's say you have a data property called "backgroundColor" in your Vue component. You want to dynamically change the background color of an element based on this property. To achieve this, you can create a computed property that returns the CSS value for the background color.
Here's how you can do it in Vue 3:
<template>
<div :style="{ backgroundColor: computedBackgroundColor }">
<!-- Your content here -->
</div>
</template>
<script>
export default {
data() {
return {
backgroundColor: 'red',
};
},
computed: {
computedBackgroundColor() {
// Add your logic to calculate the background color
// based on other data properties or conditions
return this.backgroundColor;
},
},
};
</script>
In this example, the computedBackgroundColor
computed property is used in the :style
binding to dynamically update the background color of the <div>
element. You can modify the logic inside the computed property to calculate the background color based on your specific requirements.
By using the v-bind directive with a computed property, you can easily add dynamic CSS properties to your Vue components in Vue 3.
Are computed properties only used for styling in Vue 3?
Computed properties in Vue 3 are not only used for styling; they serve a broader purpose. While computed properties are commonly used to dynamically calculate and update values for styling purposes, they can also be utilized for a variety of other tasks.Computed properties in Vue 3 are essentially methods that are cached and only re-evaluated when their dependencies change. This makes them an efficient way to perform calculations based on the data in your component.
Apart from styling, computed properties can be used for data manipulation, filtering, sorting, and even for making complex calculations based on multiple data points. For example, you can use a computed property to calculate the total price of items in a shopping cart, or to filter a list of items based on a specific condition.
By using computed properties, you can keep your component's template clean and readable, as computed properties abstract away the complex logic behind their calculations. This makes your code more maintainable and easier to understand.
In summary, while computed properties are commonly used for styling in Vue 3, they are not limited to that purpose. They offer a powerful tool for performing dynamic calculations and data manipulation in your components.