Mastering Vue 3 Composition API: A Deep Dive into Extend and Override Components
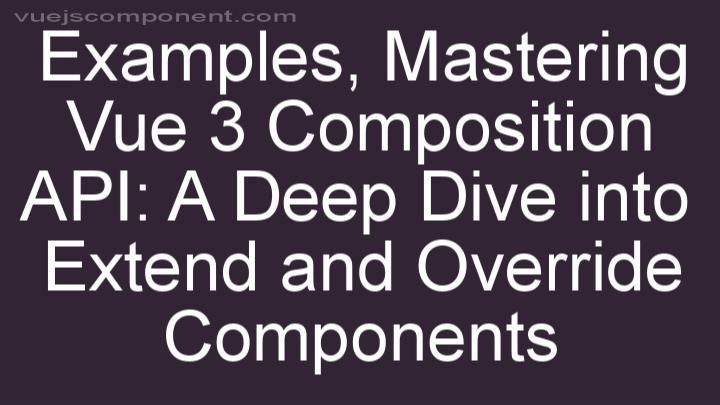
Introduction:
Welcome to the world of Vue 3 Composition API! In this blog post, we will explore one of the most powerful features of Vue 3: extending and overriding components using the Composition API. By the end of this article, you'll be equipped with the knowledge and techniques to master this aspect of Vue 3 and take your application development to the next level.
Section 1: Understanding Vue 3 Composition API
Before we dive into extending and overriding components, let's take a moment to understand what the Composition API is and how it differs from the Options API. In Vue 2, we were familiar with the Options API, which allowed us to define our component's behavior using properties, methods, and lifecycle hooks. However, the Composition API takes a different approach.
The Composition API is based on the concept of functions and reactivity. Instead of scattering our component's logic across multiple options, we can now encapsulate related logic in functions called composition functions. These functions can then be used within our component, resulting in a more organized and reusable codebase.
One of the key advantages of using the Composition API is its ability to handle complex application logic more efficiently. With the Composition API, we can easily break down our application into smaller, reusable functions, making it easier to reason about and test our code. Additionally, the Composition API promotes code reusability, as we can extract and share composition functions across multiple components.
Section 2: Extending Components with Vue 3 Composition API
In Vue 3, we have a new function called extend()
that allows us to extend existing components and add additional functionality to them. This feature is incredibly powerful, as it enables us to build on top of existing components without modifying their original behavior.
To extend a component using the Composition API, we simply create a new composition function and use the extend()
function to merge it with the existing component. This allows us to leverage the existing component's properties and methods while adding our own custom logic.
Let's say we have a Button
component that we want to extend with some additional functionality. We can create a new composition function called useExtendedButton
and use the extend()
function to merge it with the original Button
component. This way, we can maintain the original behavior of the Button
component while adding our own custom logic.
Section 3: Overriding Components with Vue 3 Composition API
There are scenarios where we may want to completely override a component's options to customize its behavior. Vue 3's Composition API provides us with the flexibility to do just that. Using reactive properties and functions, we can override a component's options and define our own custom behavior.
To override a component's options, we can create a new composition function and define our desired properties and methods. We can then use these properties and methods in our component, effectively overriding the original options.
For example, let's say we have a Modal
component that we want to override to customize its close behavior. We can create a new composition function called useCustomModal
and define a reactive property called isModalOpen
along with a method called closeModal
. We can then use these properties and methods in our component, effectively overriding the original close behavior of the Modal
component.
Section 4: Best Practices for Extending and Overriding Components
While extending and overriding components can be incredibly powerful, it's important to use them judiciously and understand the best practices associated with these techniques.
Firstly, it's crucial to understand when to use extension versus overriding based on your specific requirements. Extension is generally preferred when we want to add additional functionality to an existing component while maintaining its original behavior. On the other hand, overriding is useful when we want to completely customize a component's behavior.
Secondly, it's important to be aware of potential pitfalls and common mistakes when working with extended or overridden components. It's easy to unintentionally introduce conflicts or unintended behavior when extending or overriding components, so it's important to thoroughly test and validate your changes.
Finally, it's advisable to establish a clear organization strategy for extended and overridden components within your project. This can include creating dedicated folders or modules to house these components, making it easier to manage and maintain them in the long run.
Conclusion:
In this blog post, we have explored the power and flexibility of Vue 3's Composition API when it comes to extending and overriding components. We have seen how the Composition API can improve code organization, reusability, and allow us to build complex applications more efficiently.
By following the techniques and best practices outlined in this article, you'll be well-equipped to take advantage of the extend and override features provided by the Composition API. So go ahead, experiment with extending and overriding components in your Vue 3 projects, and unlock the full potential of this powerful framework. Happy coding!
FREQUENTLY ASKED QUESTIONS
What is the Mastering Vue 3 Composition API: A Deep Dive into Extend and Override Components course about?
The "Mastering Vue 3 Composition API: A Deep Dive into Extend and Override Components" course is a comprehensive guide that delves into the intricacies of Vue 3's Composition API, focusing specifically on extending and overriding components. This course is designed to help you master the Composition API and take your Vue development skills to the next level.Throughout the course, you will learn how to effectively utilize the Composition API to create reusable and maintainable components. You will explore the concept of extending components, allowing you to build upon existing components and add new functionality. Additionally, you will discover how to override components, enabling you to modify their behavior and appearance to suit your specific needs.
By the end of this course, you will have a deep understanding of the Composition API and be equipped with the knowledge and skills to confidently extend and override components in your Vue 3 projects. Whether you are a beginner or an experienced Vue developer, this course will provide you with invaluable insights and techniques to enhance your Vue development workflow.
What topics are covered in the course?
In the course, we cover a wide range of topics to help you enhance your skills and knowledge. Some of the main topics include:
-
Introduction to [course topic]: We start by providing an overview of the subject, its importance, and how it can benefit you.
-
Fundamentals: We delve into the fundamental concepts and principles of [course topic], giving you a solid foundation to build upon.
-
Techniques and Strategies: We explore various techniques and strategies used in [course topic], equipping you with practical tools to apply in real-life situations.
-
Case Studies: We analyze real-life case studies to demonstrate how [course topic] is applied in different scenarios. This helps you understand the practical implications and challenges faced in the field.
-
Industry Trends and Innovations: We discuss the latest trends and innovations in [course topic], keeping you up-to-date with the ever-evolving landscape.
-
Best Practices: We share proven best practices and expert tips to help you excel in [course topic], giving you a competitive edge.
-
Practical Exercises and Assignments: Throughout the course, you will have the opportunity to participate in practical exercises and assignments to apply your learning and reinforce key concepts.
-
Q&A Sessions: We offer regular Q&A sessions where you can ask questions, clarify doubts, and engage in meaningful discussions with both the instructor and fellow learners.
By covering these topics, our course aims to provide you with a comprehensive understanding of [course topic] and empower you to succeed in your endeavors.
How long does the course take to complete?
The duration of the course can vary depending on the specific program you choose. Typically, the course takes around [X] weeks to complete. However, it is important to note that the length of the course can be influenced by factors such as your availability, learning pace, and the amount of time you can dedicate to studying each week. It's always best to check the course details or contact the course provider directly for more accurate information on the duration of the specific course you are interested in.
Do I need any prerequisites to take this course?
To take this course, there are no prerequisites required. You can jump right in and start learning! Whether you're a beginner or have some prior knowledge, this course is designed to accommodate learners of all levels. So, no need to worry about any prerequisites holding you back. Just bring your enthusiasm and eagerness to learn, and you'll be all set!