Mastering Vue.draggable: A Guide to Drag and Drop Outside of a div
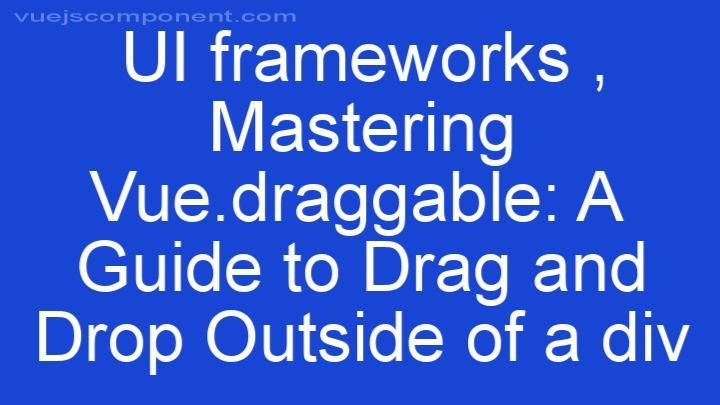
Introduction:
Drag and drop functionality has become a popular feature in web development, allowing users to interact with content in an intuitive and engaging way. Whether it's rearranging elements on a page or organizing files in a file manager, drag and drop provides a seamless user experience. However, implementing drag and drop outside of a div can be a bit trickier. That's where Vue.draggable comes in.
In this guide, we will explore the world of Vue.draggable and learn how to master the art of drag and drop outside of a div using this powerful Vue.js library. Whether you're new to Vue.js or an experienced developer looking to enhance your skills, this friendly and helpful guide will provide you with all the information you need to get started.
Section 1: Understanding Vue.draggable
Before we dive into the implementation details, let's take a moment to understand what Vue.draggable is and how it can help us create drag and drop functionality in Vue.js. Vue.draggable is a Vue.js component that enables us to easily implement drag and drop features in our web applications. It provides a simple and intuitive API that allows us to handle drag and drop events and manipulate elements on the page.
One of the key features of Vue.draggable is its flexibility. It can be used with any HTML element, not just divs. This means that we can implement drag and drop functionality on a wide range of elements, such as images, lists, and even entire sections of our page.
Section 2: Setting up Vue.draggable
Now that we have a good understanding of what Vue.draggable is, let's move on to setting it up in our project. The first step is to install Vue.draggable. Thankfully, the installation process is quite straightforward.
To install Vue.draggable, we need to add it as a dependency in our project. We can do this using npm or yarn, depending on our preference. Once we have installed Vue.draggable, we need to import it into our Vue.js component and register it as a global or local component, depending on our project structure.
In addition to the installation process, it's also important to address any common issues that might arise during setup. For example, some users might encounter compatibility issues with older versions of Vue.js or other libraries. We should provide troubleshooting tips for these scenarios to ensure a smooth setup process for our readers.
Section 3: Implementing Drag and Drop Outside of a div
Now that we have Vue.draggable set up in our project, let's explore how we can implement drag and drop functionality outside of a div. There are various scenarios where dragging elements outside of a div can be useful. For example, imagine a file manager where users can drag files from one folder to another or a Kanban board where tasks can be dragged between different columns.
To implement this feature using Vue.draggable, we need to define a draggable element and a drop zone. The draggable element is the element that the user can drag, and the drop zone is the area where the user can drop the dragged element. By handling the appropriate drag and drop events, we can manipulate the elements as desired.
In this section, we will provide detailed instructions on how to implement drag and drop outside of a div using Vue.draggable. We will include code examples and explain the underlying concepts to ensure a clear understanding for our readers.
Section 4: Advanced Techniques and Customization Options
Vue.draggable provides us with a wide range of customization options to enhance our drag and drop functionality. In this section, we will explore some advanced techniques and customization options that can take our drag and drop experience to the next level.
For example, we can restrict dragging to specific handles within the draggable element, allowing for more fine-grained control. We can also define multiple drop zones with different behaviors, such as accepting only certain types of dragged elements or triggering specific actions upon dropping.
Additionally, we will discuss event handlers that allow us to react to various drag and drop events, such as when an element is being dragged, dropped, or hovered over. These event handlers open up endless possibilities for creating interactive and dynamic user interfaces.
We will also provide tips and best practices for optimizing performance when working with large datasets or complex interactions. This includes techniques such as virtual scrolling and debouncing drag and drop events to ensure a smooth user experience.
Section 5: Troubleshooting Common Issues
While Vue.draggable is a powerful library, it's not uncommon to encounter challenges or errors during the implementation process. In this section, we will address some common issues that users may face and provide solutions or workarounds for each problem.
For example, users might struggle with handling nested draggable elements or dealing with elements that have dynamic content. We will provide step-by-step solutions and explain the underlying reasons for these issues, ensuring that our readers feel supported throughout their implementation process.
Conclusion:
Congratulations! You have successfully mastered Vue.draggable and learned how to implement drag and drop functionality outside of a div using this fantastic Vue.js library. We hope this comprehensive guide has provided you with the knowledge and confidence to create engaging and interactive web applications.
Remember, practice makes perfect. Don't hesitate to experiment with your own projects and explore further resources to expand your skills. Vue.draggable is just the tip of the iceberg when it comes to creating dynamic and user-friendly interfaces. Happy coding!
FREQUENTLY ASKED QUESTIONS
Why should I use Vue.draggable?
There are several reasons why you should consider using Vue.draggable. Firstly, Vue.draggable is a powerful and flexible library that allows you to easily implement drag-and-drop functionality in your Vue.js applications. This can greatly enhance the user experience and make your application more interactive.One of the key benefits of Vue.draggable is its simplicity. It provides a straightforward API that is easy to understand and use, even for beginners. You don't need to worry about complex configuration or setup processes. With just a few lines of code, you can enable drag-and-drop functionality in your application.
Another advantage of Vue.draggable is its versatility. It supports various types of draggable elements, such as lists, grids, and tables. This means you can use it in a wide range of scenarios, from creating sortable lists to building advanced user interfaces.
Vue.draggable also offers a high level of customization. You have full control over the appearance and behavior of the draggable elements. You can define custom drag handles, specify the axis of movement, and even implement custom animations. This allows you to tailor the drag-and-drop experience to match the unique requirements of your application.
Furthermore, Vue.draggable integrates seamlessly with Vue.js, which is a popular JavaScript framework for building user interfaces. It follows the reactive programming paradigm of Vue.js, which means any changes to the draggable elements are automatically reflected in the underlying data. This makes it easy to keep track of the order and position of the draggable elements.
In summary, using Vue.draggable can greatly enhance the interactivity and usability of your Vue.js applications. Its simplicity, versatility, customization options, and seamless integration with Vue.js make it a powerful tool for implementing drag-and-drop functionality. So, if you want to take your application to the next level, give Vue.draggable a try!
Can I use Vue.draggable outside of a div?
Yes, you can use Vue.draggable outside of a div. Vue.draggable is a powerful component that allows you to create draggable elements within a container, but it is not limited to only being used within a div. You can use it within any HTML element that supports events, such as a section, article, or even the body of your webpage.To use Vue.draggable outside of a div, you need to make sure you have included the Vue.draggable library in your project. Once you have done that, you can simply wrap your desired element with the Vue.draggable component. This will enable the element to be draggable.
Here's an example of how you can use Vue.draggable outside of a div:
<template>
<section>
<h1>Draggable Element</h1>
<VueDraggable>
<p>This paragraph is draggable!</p>
</VueDraggable>
</section>
</template>
<script>
import VueDraggable from 'vue-draggable';
export default {
components: {
VueDraggable,
},
};
</script>
In this example, we have wrapped a <p>
element with the VueDraggable component inside a <section>
. This will make the paragraph draggable within the section. You can customize the behavior and appearance of the draggable element by using the various props and events provided by Vue.draggable.
Remember to check the documentation of Vue.draggable for more details and options on how to use it effectively outside of a div. Happy dragging!
What are the benefits of dragging and dropping outside of a div?
Dragging and dropping outside of a div can offer several benefits. Firstly, it allows for greater flexibility in organizing and rearranging elements on a webpage. By dragging and dropping outside of a specific container, you have the freedom to place elements anywhere on the page, rather than being limited to the confines of a single div.Additionally, dragging and dropping outside of a div can enhance the user experience by providing a more intuitive and interactive interface. Users can simply click and drag objects to their desired location, eliminating the need for complex navigation or input methods.
Moreover, this technique can improve the overall aesthetics of a webpage. By allowing elements to be positioned freely, you can create visually appealing layouts and designs. This can be particularly useful for creating responsive and dynamic websites.
Furthermore, dragging and dropping outside of a div can facilitate better collaboration and productivity. It enables users to easily move and share content between different sections or areas of a webpage, making it convenient for tasks such as content management or file organization.
In summary, the benefits of dragging and dropping outside of a div include increased flexibility in organizing elements, improved user experience, enhanced aesthetics, and better collaboration.
Is Vue.draggable compatible with other frameworks?
Yes, Vue.draggable is compatible with other frameworks. It is built on top of the Vue.js framework and can be used alongside other frameworks such as React, Angular, and jQuery. The flexibility and versatility of Vue.draggable allow it to seamlessly integrate into various project setups, making it a great choice for developers working with different frameworks. Whether you're building a single-page application or a complex web project, Vue.draggable can be easily incorporated to enhance the drag-and-drop functionality. So, go ahead and explore the possibilities of combining Vue.draggable with your preferred framework!