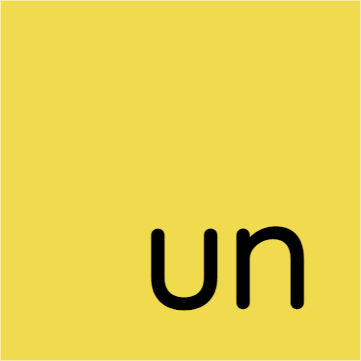
Install
unenv
unenv
is a framework-agnostic system that allows converting JavaScript code to be platform agnostic and work in any environment including Browsers, Workers, Node.js, or JavaScript runtime.
Install
# Using npm
npm i -D unenv
# Using yarn
yarn add --dev unenv
# Using pnpm
pnpm add -D unenv
Usage
Using env
utility and built-in presets, unenv
will provide an abstract configuration that can be used in building pipelines (rollup.js, webpack, etc.).
import { env } from "unenv";
const { alias, inject, polyfill, external } = env({}, {}, {});
Note: You can provide as many presets as you want. unenv will merge them internally and the right-most preset has a higher priority.
Presets
node
Suitable to convert universal libraries working in Node.js.
- Add supports for global
fetch
API - Set Node.js built-ins as externals
import { env, nodeless } from "unenv";
const envConfig = env(node, {});
nodeless
Suitable to transform libraries made for Node.js to run in other JavaScript runtimes.
import { env, nodeless } from "unenv";
const envConfig = env(nodeless, {});
deno
This preset can be used to extend nodeless
to use Deno's Node.js API Compatibility (docs, docs).
[!WARNING]
This preset is experimental and behavior might change!
import { env, nodeless, deno } from "unenv";
const envConfig = env(nodeless, deno, {});
cloudflare
This preset can be used to extend nodeless
to use Cloudflare Worker Node.js API Compatibility (docs).
[!WARNING]
This preset is experimental and behavior might change!
[!NOTE]
Make sure to enablenodejs_compat
compatibility flag.
import { env, nodeless, cloudflare } from "unenv";
const envConfig = env(nodeless, cloudflare, {});
vercel
This preset can be used to extend nodeless
to use Vercel Edge Node.js API Compatibility (docs).
[!WARNING]
This preset is experimental and behavior might change!
import { env, nodeless, vercel } from "unenv";
const envConfig = env(nodeless, vercel, {});
Built-in Node.js modules
unenv
provides a replacement for all Node.js built-ins for cross-platform compatibility.
npm packages
unenv
provides a replacement for common npm packages for cross platform compatibility.
Package | Status | Source |
---|---|---|
npm/consola | Use native console |
unenv/runtime/npm/consola |
npm/cross-fetch | Use native fetch |
unenv/runtime/npm/cross-fetch |
npm/debug | Mocked with console.debug |
unenv/runtime/npm/debug |
npm/fsevents | Mocked | unenv/runtime/npm/fsevents |
npm/inherits | Inlined | unenv/runtime/npm/inherits |
npm/mime-db | Minimized | unenv/runtime/npm/mime-db |
npm/mime | Minimized | unenv/runtime/npm/mime |
npm/node-fetch | Use native fetch |
unenv/runtime/npm/node-fetch |
npm/whatwg-url | Use native URL |
unenv/runtime/npm/whatwg-url |
Auto-mocking proxy
import MockProxy from "unenv/runtime/mock/proxy";
console.log(MockProxy().foo.bar()[0]);
The above package doesn't work outside of Node.js and neither we need any platform-specific logic! When aliasing os
to mock/proxy-cjs
, it will be auto-mocked using a Proxy Object which can be recursively traversed like an Object
, called like a Function
, Iterated like an Array
, or instantiated like a Class
.
We use this proxy for auto-mocking unimplemented internals. Imagine a package does this:
const os = require("os");
if (os.platform() === "windows") {
/* do some fix */
}
module.exports = () => "Hello world";
By aliasing os
to unenv/runtime/mock/proxy-cjs
, the code will be compatible with other platforms.
Other polyfills
To discover other polyfills, please check ./src/runtime.
License
MIT