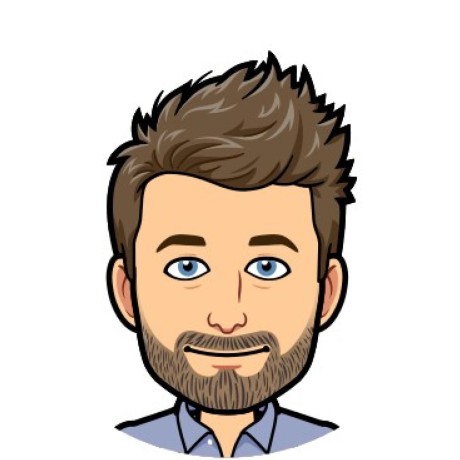
Install
Vue Mixin Decorator
This library fully depends on vue-class-component.
Most ideas and code are stolen borrowed from @HerringtonDarkholme
and his av-ts project. Also from
@JsonSong89's
comment, who suggested that the idea
should be extracted into a separate project which is why I've begrudgingly done so.
Project template shamelessly stolen from vue-property-decorator.
Best case scenario is this project/implementation/concept
gets merged/provided into/by an officially supported project
and this one can be deprecated.
License
MIT License
Install
npm install --save-dev vue-mixin-decorator
Usage
There are 2 decorators:
@Mixin
@Component
and an extension class:
Mixins
Note: @Mixin
is @Component
exported from vue-class-component
.
Single Mixin
import Vue from 'vue';
import { Component, Mixin, Mixins } from 'vue-mixin-decorator';
@Mixin
class MyMixin extends Vue {
created() {
console.log('Mixin created()');
}
mixinMethod() {
console.log('Mixin method called.');
}
}
@Component
class MyComponent extends Mixins<MyMixin>(MyMixin) {
created() {
this.mixinMethod();
}
}
Multiple Mixins
import Vue from 'vue';
import { Component, Mixin, Mixins } from 'vue-mixin-decorator';
@Mixin
class MyMixin extends Vue {
created() {
console.log('Mixin created()');
}
mixinMethod() {
console.log('Mixin method called.');
}
}
@Mixin
class MyOtherMixin extends Vue {
created() {
console.log('Other mixin created()');
}
otherMixinMethod() {
console.log('Other mixin method called.');
}
}
// Create an interface extending the mixins to provide
interface IMixinInterface extends MyMixin, MyOtherMixin {}
@Component
class MyComponent extends Mixins<IMixinInterface>(MyMixin, MyOtherMixin) {
created() {
this.mixinMethod();
this.otherMixinMethod();
}
}