Solving Type Errors Like a Pro: A Deep Dive into Typing Props with Interfaces in Vue 3
Introduction:
Section 1: Understanding the Basics of Typing Props in Vue 3
I. This utility allows us to specify the expected type of a prop, such as a string, number, or boolean. Let's take a look at an example:
Section 2: Introducing Interfaces for Typing Props
Section 3: Solving Common Type Errors with Interfaces
1. Type 'X' is not assignable to type 'Y':
2. Property 'X' does not exist on type 'Y':
3. Object is possibly 'null' or 'undefined':
Section 4: Best Practices for Using Interfaces and Typing Props in Vue 3
1. Organize and structure interfaces for readability:
2. Handle optional props, default values, and required props with care:
3. Choose between inline type annotations and interfaces wisely:
Conclusion:
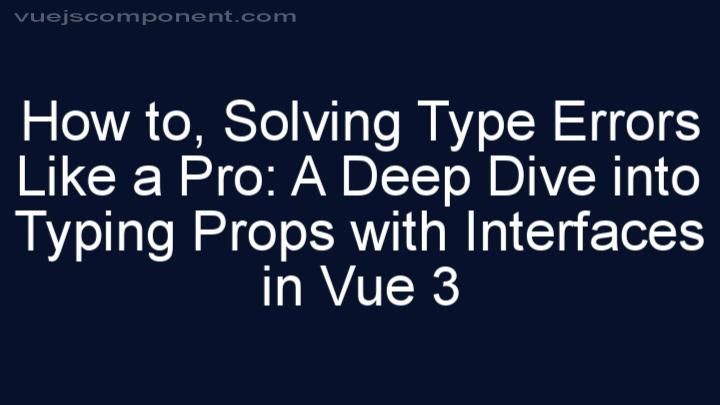
Introduction:
Welcome, Vue 3 developers! In today's blog post, we're going to delve deep into the world of typing props with interfaces in Vue 3. As you may know, type checking is a crucial aspect of modern web development, as it helps prevent errors and enhances code maintainability. In this article, we will explore the power of interfaces in Vue 3 and how they can help us solve type errors effectively.
Section 1: Understanding the Basics of Typing Props in Vue 3
Before we dive into the specifics of interfaces, let's first establish a solid understanding of props in Vue. In Vue, props are a way for components to communicate with each other. They allow data to flow from a parent component to a child component, enabling a modular and reusable approach to building user interfaces.
Now, you might be wondering, why do we need to type props? Well, typing props provides several benefits. Firstly, it improves code maintainability by making it clear what type of data a component expects. This makes it easier for developers to reason about the codebase and reduces the chances of introducing bugs. Secondly, type checking provides early error detection, allowing you to catch potential issues before they manifest at runtime.
In Vue 3, we can leverage TypeScript to define prop types. TypeScript is a statically typed superset of JavaScript that provides additional tooling for type checking. By using TypeScript in Vue 3, we can ensure that our props are correctly typed, leading to more robust and reliable code.
To define prop types in Vue 3, we use the PropType
utility provided by the Vue composition
AP
I. This utility allows us to specify the expected type of a prop, such as a string, number, or boolean. Let's take a look at an example:
import { defineComponent, PropType } from 'vue';
export default defineComponent({
props: {
message: {
type: String as PropType<string>,
required: true,
},
count: {
type: Number as PropType<number>,
default: 0,
},
isActive: {
type: Boolean as PropType<boolean>,
default: false,
},
},
});
In this example, we define three props: message
, count
, and isActive
. Each prop has a specified type using the PropType
utility, ensuring that the prop values adhere to the expected types. The required
and default
options allow us to specify whether a prop is mandatory or has a default value.
Section 2: Introducing Interfaces for Typing Props
Now that we have a solid understanding of typing props using the PropType
utility, let's explore how interfaces can take our prop typing game to the next level. In TypeScript, interfaces are a powerful tool for defining the shape of an object. They allow us to describe the structure and types of the properties in an object, providing a clear contract for usage.
When it comes to typing props in Vue 3, interfaces can be used to define the expected shape of a prop object. Instead of inline type annotations, interfaces offer a more organized and reusable approach to prop typing. By defining interfaces for props, we can encapsulate the expected prop structure and reuse it across multiple components.
Using interfaces for prop typing offers several advantages. Firstly, it improves code readability and maintainability. By defining the expected prop structure in an interface, it becomes easier to understand the props a component expects. Additionally, interfaces promote code reuse by allowing us to define common prop structures that can be shared across multiple components. This reduces duplication and makes our codebase more modular.
Let's take a look at an example of how we can use interfaces to type props in Vue 3:
import { defineComponent } from 'vue';
interface MessageProps {
message: string;
count: number;
isActive: boolean;
}
export default defineComponent({
props: {
message: {
type: String as PropType<MessageProps['message']>,
required: true,
},
count: {
type: Number as PropType<MessageProps['count']>,
default: 0,
},
isActive: {
type: Boolean as PropType<MessageProps['isActive']>,
default: false,
},
},
});
In this example, we define an interface called MessageProps
that describes the shape of our props. We then use the interface to specify the prop types, ensuring that the props adhere to the defined structure. By using interfaces, we can easily reuse the MessageProps
interface across multiple components, promoting code reuse and maintainability.
Section 3: Solving Common Type Errors with Interfaces
As developers, we often encounter type errors when working with props in Vue 3. These errors can be frustrating and time-consuming to debug. However, by leveraging interfaces, we can effectively solve these type errors and improve our development experience.
Let's identify and explore some common type errors that developers may encounter when working with props in Vue 3:
1. Type 'X' is not assignable to type 'Y':
This error typically occurs when the expected type of a prop does not match the actual type provided. To solve this error, ensure that the prop is correctly typed in the interface and that the assigned value adheres to the defined type.
2. Property 'X' does not exist on type 'Y':
This error occurs when accessing a property on a prop that does not exist in its defined interface. To solve this error, double-check the prop's interface definition and make sure the accessed property is present.
3. Object is possibly 'null' or 'undefined':
This error can occur when accessing properties on optional props. To solve this error, use optional chaining (prop?.property
) or provide a default value for the prop.
By leveraging interfaces, we can effectively solve these type errors. Interfaces provide a clear contract for the expected prop structure, allowing us to catch errors early and ensure type safety in our code.
Section 4: Best Practices for Using Interfaces and Typing Props in Vue 3
To wrap up our deep dive into typing props with interfaces in Vue 3, let's discuss some best practices that can enhance our prop typing experience:
1. Organize and structure interfaces for readability:
When defining interfaces for props, aim for clarity and organization. Group related props together and use descriptive names for interfaces to make it easier for other developers to understand the expected prop structure.
2. Handle optional props, default values, and required props with care:
When working with optional props, use the PropType<Prop | undefined>
syntax to indicate that the prop can be undefined. For default values, use the default
option in the prop definition. For required props, set the required
option to true
in the prop definition.
3. Choose between inline type annotations and interfaces wisely:
While interfaces offer a more organized and reusable approach to prop typing, inline type annotations can be useful in certain scenarios. Use inline type annotations when the prop structure is simple and doesn't require reuse across multiple components. For complex prop structures, interfaces are the way to go.
Conclusion:
Congratulations, Vue 3 developers! We have explored the power of typing props with interfaces and learned how they can help us solve type errors effectively. By leveraging interfaces, we can improve code maintainability, catch errors early, and promote code reuse. Remember to apply these techniques in your own projects for improved code quality and error prevention.
If you're hungry for more knowledge on advanced prop typing techniques in Vue 3, be sure to check out the official Vue documentation and other online resources. Happy coding!
FREQUENTLY ASKED QUESTIONS
Why is typing props important in Vue 3?
Typing props in Vue 3 is important for several reasons. First, it helps to improve the overall code quality and maintainability of your Vue components. By defining the types of props, you can catch any potential errors or inconsistencies early on, which can save you time troubleshooting later.Secondly, typing props provides better documentation and understanding of the component's interface. When other developers or team members work with your component, they can easily see what props are available and what type of data is expected. This makes collaboration and code reuse much smoother.
Additionally, typing props allows for better tooling support. With proper type annotations, you can take advantage of features like auto-completion, type checking, and IDE suggestions. This can greatly enhance your development workflow and help prevent common mistakes.
Lastly, by typing props, you can catch potential bugs and errors during the development process rather than discovering them in runtime. This can lead to more robust and reliable code.
Overall, typing props in Vue 3 is a recommended practice that can improve code quality, documentation, collaboration, and tooling support. It's a small investment upfront that can lead to significant benefits in the long run.
How can I implement interfaces for typing props in Vue 3?
To implement interfaces for typing props in Vue 3, you can utilize TypeScript, which is fully supported in Vue
3. TypeScript allows you to define types for your props, providing better type checking and documentation for your components.To begin, you'll need to install TypeScript in your Vue 3 project. You can do this by running the following command in your project directory:
npm install --save-dev typescript
Once TypeScript is installed, rename your .js
files to .ts
files. This will enable TypeScript support in your Vue project.
Next, create an interface for your props by defining the type of each prop. For example, let's say you have a component called MyComponent
with two props: name
of type string
and age
of type number
. You can define an interface for these props like this:
interface MyComponentProps {
name: string;
age: number;
}
Now, in your component options, specify the prop types using the Props
option and assign the interface you created as the type:
import { defineComponent, PropType } from 'vue';
export default defineComponent({
props: {
name: {
type: String as PropType<MyComponentProps['name']>,
required: true,
},
age: {
type: Number as PropType<MyComponentProps['age']>,
required: true,
},
},
// ... rest of the component options
});
By using TypeScript and defining the prop types in this way, you will benefit from improved type checking and better documentation for your props.
Remember to also update the places where you use MyComponent
and pass the props accordingly, ensuring that the types match.
I hope this helps! Let me know if you have any further questions.
Can you provide an example of using interfaces for typing props in Vue 3?
Certainly! In Vue 3, you can use interfaces to type props by utilizing the defineComponent
function. This allows you to define the structure and type of props that your component expects.Here's an example of how you can use interfaces for typing props in Vue 3:
import { defineComponent } from 'vue';
interface MyComponentProps {
name: string;
age: number;
}
const MyComponent = defineComponent({
props: {
name: {
type: String,
required: true
},
age: {
type: Number,
required: true
}
},
setup(props: MyComponentProps) {
// Access props using the defined interface
console.log(props.name);
console.log(props.age);
// Rest of the component's setup logic
}
});
export default MyComponent;
In this example, we define an interface MyComponentProps
that specifies the name
prop as a string and the age
prop as a number. Inside the defineComponent
function, we use the props
option to define the props and their types.
By using the setup
function, we can access the props using the defined interface. This provides type checking and helps ensure that the props are used correctly within the component.
Remember to import the defineComponent
function from the Vue package and export your component for it to be used in your application.
I hope this example helps you understand how to use interfaces for typing props in Vue 3. Let me know if you have any further questions!
What happens if the passed prop doesn't match the defined interface?
If the passed prop doesn't match the defined interface, it can lead to potential issues in your code. When using interfaces in TypeScript, they act as a contract that defines the expected shape of an object. If the passed prop doesn't adhere to this contract, you may encounter unexpected behavior or errors.One possible consequence is that your code might fail at runtime. TypeScript is a statically typed language, which means it performs type checking at compile-time. If the actual type of the prop doesn't match the expected type defined in the interface, TypeScript will raise a type error during compilation. This error will indicate that the prop is incompatible with the interface, and you'll need to fix the issue before your code can run successfully.
Another consequence is that you may encounter bugs or logical errors in your application. If the prop doesn't have the required properties or methods defined in the interface, your code might break or behave unexpectedly when accessing those properties or invoking those methods. This can lead to hard-to-debug issues and potentially impact the overall functionality of your application.
To avoid these problems, it's important to ensure that the passed prop matches the defined interface. Double-check the types and structure of your props to ensure they align with the interface expectations. Additionally, consider using TypeScript's type assertions or runtime checks to validate the shape of the props before using them in your code.
By properly aligning the passed prop with the defined interface, you can ensure the smooth functioning of your code and reduce the chances of errors or bugs.