The Ultimate Guide to Global Validators in Vuetify 3 and Vee-Validate: Everything You Need to Know
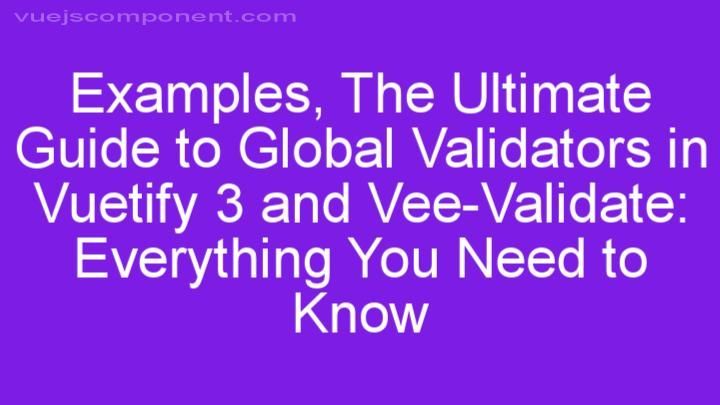
Introduction:
Welcome readers to the ultimate guide on global validators in Vuetify 3 and Vee-Validate. In the world of web development, validators play a crucial role in ensuring data integrity and providing a smooth user experience. They help validate user input, enforce data validation rules, and prevent incorrect or invalid data from being submitted.
Section 1: Understanding Global Validators
Global validators, as the name suggests, are validators that can be accessed and utilized throughout your entire application. They offer a centralized approach to form validation, allowing you to define validation rules once and apply them across multiple forms or components.
In Vuetify 3 and Vee-Validate, global validators can be defined and configured in a straightforward manner. By defining them at a global level, you ensure that the same validation rules are applied consistently across all instances of a particular form or component.
Global validators can be used to enforce common validation rules such as required fields, email formats, password strength, and more. They provide a convenient and efficient way to maintain consistency and accuracy in your form submissions.
Section 2: Getting Started with Vuetify 3 Validators
Vuetify 3 introduces several new features and improvements in the realm of validators. To get started with Vuetify 3 validators, you need to set up and configure them correctly.
First, make sure you have Vuetify 3 installed in your project. Once that is done, you can start by adding the necessary dependencies for validation. Vuetify 3 provides a variety of validation rules out of the box, including required, min/max length, email, and more.
To implement validation rules, you can take advantage of Vuetify's v-model
directive and the rules
prop. By binding the rules
prop to an array of validation rules, you can enforce specific validation criteria on your form fields. Vuetify 3 also supports validation messages, allowing you to display custom error messages based on different validation scenarios.
In this section, we'll provide you with code snippets and step-by-step instructions on implementing different types of validation rules using Vuetify 3. Whether you're validating a simple text input or a complex form with multiple fields, you'll find all the information you need to get started with Vuetify 3 validators.
Section 3: Exploring Advanced Validation Techniques with Vee-Validate
While Vuetify 3 provides a solid foundation for form validation, there are cases where you may need more advanced validation techniques. This is where Vee-Validate comes into play.
Vee-Validate is a powerful validation library that can be seamlessly integrated with Vuetify 3. It offers additional features such as custom error messages, asynchronous validations, conditional validations, and more. With Vee-Validate, you can take your form validation to the next level and handle complex validation scenarios with ease.
To get started with Vee-Validate, you'll need to install it as a dependency in your project. Once installed, you can integrate Vee-Validate with Vuetify 3 by using its validation functions and directives. Vee-Validate provides a comprehensive set of validation rules and methods that can be used to define complex validation logic.
In this section, we'll explore advanced validation techniques using Vee-Validate. We'll cover topics such as custom error messages, asynchronous validations (e.g., validating data against an API), and conditional validations (e.g., validating a field based on the value of another field). By the end of this section, you'll have a solid understanding of how to leverage Vee-Validate to handle advanced form validation needs.
Section 4: Leveraging Global Validators for Consistency
Consistency is key when it comes to form validation. It's important to ensure that the same validation rules are applied consistently across your entire application. This not only improves the user experience but also helps maintain data integrity and accuracy.
Global validators provide a powerful tool for achieving consistency in form validation. By defining validation rules at a global level, you can ensure that they are applied uniformly throughout your project. This eliminates the need to repeat the same validation logic across multiple forms or components, saving you time and effort.
In this section, we'll discuss how you can leverage global validators to achieve consistency in your application. We'll share tips and best practices for organizing and managing global validators effectively. Additionally, we'll explore how global validators can be used in conjunction with Vee-Validate to create a comprehensive and consistent validation strategy.
Section 5: Troubleshooting Common Validator Issues
While validators are designed to simplify form validation, there may be instances where you encounter challenges or issues. It's important to be prepared for such scenarios and know how to troubleshoot common validator problems.
In this section, we'll identify common challenges developers may face when working with global validators. We'll provide solutions and workarounds for these issues, including debugging techniques. Whether you're dealing with validation errors, incorrect error messages, or conflicts between different validation libraries, we'll help you navigate through these challenges and ensure a smooth validation process.
Conclusion:
In this comprehensive guide, we have covered everything you need to know about global validators in Vuetify 3 and Vee-Validate. From understanding the concept of global validators to implementing advanced validation techniques, you now have the knowledge and tools to create robust and consistent form validation in your web applications.
We encourage you to explore further resources related to form validation and Vuetify 3 to deepen your understanding and enhance your skills. As always, thank you for choosing our guide, and we remain committed to supporting your development journey. Happy coding!
FREQUENTLY ASKED QUESTIONS
Vee-Validate provides a plugin that can be installed and used alongside Vuetify 3 to enhance form validation capabilities.
Vee-Validate offers a convenient plugin that can be easily installed and utilized with Vuetify 3 to bolster the capabilities of form validation. With this plugin, you can take your form validation to the next level, ensuring that user input is accurate and meets your specified criteria. By integrating Vee-Validate into your Vuetify 3 project, you can streamline the validation process and provide a better user experience.
You can refer to the official documentation for both libraries, explore online tutorials, or join developer communities to get help and insights from other users.
To find assistance and gain insights for both libraries, you have a few options. First, you can refer to the official documentation provided by the developers. This will give you a comprehensive understanding of the libraries' features and functionalities. Additionally, you can explore online tutorials that are specifically tailored to these libraries. These tutorials often provide step-by-step instructions and examples, making it easier for you to grasp the concepts and apply them to your own projects.
Another great way to get help and insights is by joining developer communities. These communities are filled with experienced users who are willing to share their knowledge and offer assistance. You can ask questions, participate in discussions, and learn from the experiences of others.
Remember, by utilizing these resources, you'll be able to enhance your understanding of the libraries and make the most out of your development journey. Happy coding!
If you're using an older version of Vue.js, you might need to refer to the documentation or community resources to ensure compatibility or consider upgrading to Vue.js 3 for the best experience with Vuetify
If you're currently using a version of Vue.js that is not the latest, it's recommended to consult the documentation or community resources for guidance on compatibility. Alternatively, you may want to consider upgrading to Vue.js 3 to ensure the best experience when using Vuetify.
How do I use global validators in Vuetify 3?
To use global validators in Vuetify 3, you can follow these steps:
1. First, import the required validator from the Vuelidate library. You can do this by adding the following line of code at the top of your script section:
import { required } from 'vuelidate/lib/validators';
2. Next, create a global mixin to define your custom validation rules. You can do this by adding the following code:
Vue.mixin({
validations: {
$v: {
// Add your validation rules here
}
}
});
This will make the validation rules available to all components in your application.
3. Now, you can add the validation rules to your component. In the validations
section of your component, add the desired validation rule using the $each
property. For example, if you want to make a text field required, you can add the following code:
validations: {
$each: {
myTextField: {
required
}
}
}
Replace myTextField
with the name of your actual text field.
4. Finally, you can display the validation error message in your template. You can do this by using the $dirty
and $error
properties provided by Vuelidate. Here's an example:
<div>
<input v-model="$v.myTextField.$model" type="text">
<span v-if="$v.myTextField.$dirty && !$v.myTextField.required" class="error">This field is required</span>
</div>
Make sure to replace myTextField
with the actual name of your text field.
That's it! With these steps, you can use global validators in Vuetify 3 and apply them to your components. Remember to import the necessary validator, create a global mixin, define the validation rules, and display the error messages accordingly.