Troubleshooting Common Errors When Typing Props Using Interface in Vue 3
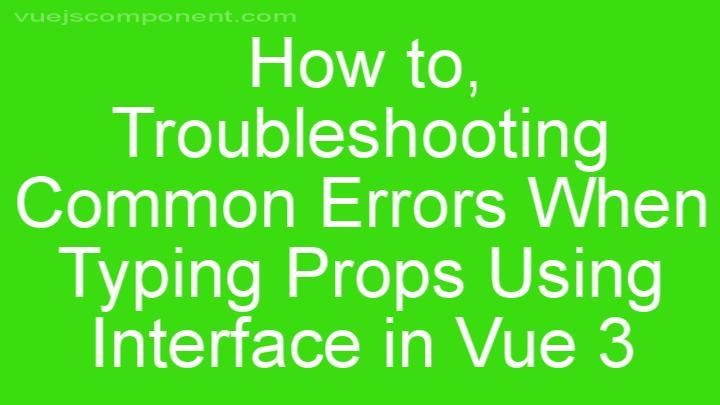
Introduction:
Hello there, Vue 3 enthusiasts! Today, we're diving into the world of props and interfaces in Vue 3. As you may already know, typing props is an essential aspect of Vue 3 development. It not only improves code quality and maintainability but also helps us catch errors early on. So, if you've encountered any issues while typing your props, fear not! We're here to guide you through the troubleshooting process and get you back on track. Let's get started!
I. Understanding Props and Interfaces in Vue 3
Before we jump into troubleshooting, let's make sure we're on the same page regarding props and interfaces in Vue 3.
Props, in Vue 3, are a way for components to communicate with each other. They allow data to flow from a parent component to its child components. By defining prop types, we can ensure that the data received is of the expected type, preventing potential bugs and enhancing code readability.
Now, let's talk about interfaces. In the context of Vue 3, interfaces are used to define the types of props. They act as blueprints, specifying the expected shape and types of the props that a component can receive. By using interfaces, we can leverage the power of TypeScript to enforce type safety and catch errors at compile-time.
Using interfaces to type props brings numerous benefits. Firstly, it provides clear documentation for both developers and future maintainers of the codebase. Secondly, it helps us catch errors early on, preventing runtime issues. Lastly, it improves code maintainability by making it easier to understand and modify components.
II. Common Errors When Typing Props
Now that we have a solid understanding of props and interfaces, let's explore some common errors that you might encounter while typing props in Vue 3.
- Error: "Property 'x' does not exist on type 'ComponentOptions'"
This error message typically occurs when you're trying to access a prop that hasn't been properly defined in the interface or component options. To troubleshoot this issue, follow these steps:
- Double-check the prop name in your template and make sure it matches the defined prop name in the interface.
- Verify that the interface defining the prop types is properly imported in your component.
- Pay attention to any typos or case sensitivity issues that may be causing the error.
By following these troubleshooting steps, you should be able to identify and rectify the issue causing the "Property 'x' does not exist on type 'ComponentOptions'" error.
- Error: "Property 'y' is missing in type '{ }'"
This error message occurs when a required prop is not provided to a component. To resolve this error, consider the following suggestions:
- Ensure that the required prop is correctly defined as a required field in the interface.
- Verify that the parent component is passing the required prop to the child component.
- If the prop is conditionally required, use Vue's
v-if
orv-show
directives to handle the case when the prop is not available.
By double-checking prop names, ensuring proper prop passing, and handling conditional requirements, you can overcome the "Property 'y' is missing in type '{ }'" error.
III. Dealing with Type Mismatch Errors
Type mismatch errors can occur when you assign an incorrect type to a prop. Let's explore a couple of common type mismatch errors and how to resolve them.
- Error: "Type '(value: string) => void' is not assignable to type 'PropType
'"
This error message indicates that you're assigning a function type to a prop that expects a number. To resolve this error, consider the following steps:
- Make sure the prop's interface or component options specify the correct type for the prop, in this case,
number
. - Verify that you're passing the prop with the correct type from the parent component.
- If the prop can accept multiple types, use union types (
number | string
, for example) in the prop's type definition.
By ensuring the correct types are assigned and resolving any type mismatches, you can overcome the "Type '(value: string) => void' is not assignable to type 'PropType
- Error: "Property 'z' has no initializer and is not definitely assigned"
This error typically occurs when you haven't assigned a value to an optional prop. To resolve this error, consider the following solutions:
- Initialize the optional prop with a default value of
null
orundefined
in the interface or component options. - Use the "!" operator to assert non-nullability if you're confident that the prop will always be assigned before being accessed.
By initializing optional props or asserting non-nullability, you can overcome the "Property 'z' has no initializer and is not definitely assigned" error.
IV. Additional Tips for Smooth Prop Typing
To make your prop typing experience even smoother, here are some additional tips and best practices:
- Provide clear and concise documentation for your components, explaining the purpose and expected props.
- Group related props together using interfaces to enhance code organization and readability.
- Leverage TypeScript's built-in utility types, such as
Partial
,Required
, andReadonly
, to handle common scenarios without reinventing the wheel.
By following these tips, you can ensure that your prop typing is effective and maintainable.
Conclusion:
Congratulations on making it through this troubleshooting guide for common errors when typing props using interfaces in Vue 3! We've covered the importance of prop typing, the role of interfaces, and how to troubleshoot common errors. Remember, even experienced developers encounter errors from time to time, but with the right knowledge and perseverance, you can overcome any obstacle.
Keep exploring Vue 3's prop typing capabilities, applying the troubleshooting tips we discussed, and never hesitate to seek help from the Vue community. Happy coding!
FREQUENTLY ASKED QUESTIONS
What is the purpose of this tutorial?
The purpose of this tutorial is to provide you with a comprehensive understanding of the topic at hand. By breaking down complex concepts into simple and easy-to-follow steps, our goal is to empower you with the knowledge and skills necessary to master the subject. Whether you're a beginner or looking to enhance your existing expertise, this tutorial aims to cater to your needs and help you achieve your learning goals. So, get ready to dive in and explore the fascinating world of [topic] with us!
It provides step-by-step guidance to resolve these issues and ensure smooth development.
Our step-by-step guidance is designed to help you resolve these issues and ensure a smooth development process. We provide clear instructions and support to guide you through each step, making it easier for you to overcome any challenges and achieve your goals. With our assistance, you can navigate through the complexities and complexities of the development process with confidence and ease.
Why am I getting a Property is missing in type error?
The "Property is missing in type" error usually occurs when you are trying to access a property that does not exist on a specific type or object. This error is commonly encountered in programming languages such as JavaScript or TypeScript.To resolve this error, you need to double-check the code and ensure that the property you are trying to access actually exists on the type or object. Make sure that you have spelled the property name correctly and that it is declared or defined properly.
If you are using TypeScript, you can also benefit from static type checking. TypeScript can help you catch such errors during development by providing type annotations and checking for type compatibility.
In some cases, this error may also occur if the property you are trying to access is optional or could be undefined. In such situations, you can use conditional checks, like the "if" statement or the optional chaining operator (?.), to handle cases where the property might not exist or be undefined.
By carefully reviewing your code and ensuring that the property you are accessing is present and properly defined, you can usually resolve the "Property is missing in type" error and prevent it from occurring in the future.
How can I fix the Property is missing in type error?
To fix the "Property is missing in type" error, you will need to carefully review your code and ensure that you have defined all the required properties in your type declaration.This error typically occurs when you are working with TypeScript and you are trying to assign a value to a variable that does not match the expected type. TypeScript is a statically typed language, which means it requires you to explicitly declare the types of your variables.
Here are a few steps you can take to resolve this error:
-
Check the type declaration: Look for the line of code where the error is being thrown and examine the type declaration for that variable. Make sure all the required properties are included in the type definition.
-
Verify the assignment: Double-check the code where you are assigning a value to the variable. Ensure that the assigned value matches the expected type and includes all the necessary properties.
-
Update the type declaration: If you find that there are missing properties in the type declaration, update it to include those properties. This will align the expected type with the actual value you are assigning.
-
Fix any other related errors: Sometimes, the "Property is missing in type" error can be a cascading effect of other errors in your code. Fix any other errors that are reported and recheck if the error still persists.
By following these steps and ensuring that your type declarations and assignments are aligned, you should be able to resolve the "Property is missing in type" error. If you are still facing issues, feel free to provide more specific details or code snippets, and I'll be happy to assist you further.