Troubleshooting Props in Vue 3: Tips and Tricks for Smooth Development
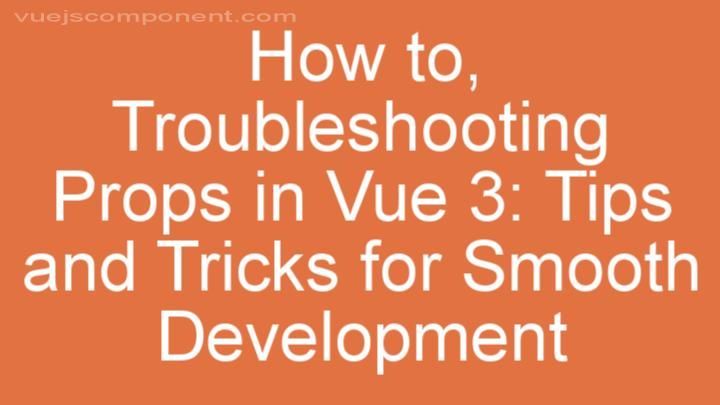
Introduction:
Props are an integral part of Vue 3 development, allowing for efficient communication between parent and child components. In this blog post, we will delve into the world of props, exploring their significance and the benefits they bring to the table.
I. Understanding Props in Vue 3:
A. What are Props?
Props, short for properties, serve as a means of passing data from parent components to child components in Vue 3. By utilizing props, we can create reusable and modular components, enhancing the overall maintainability and scalability of our Vue applications.
B. Declaring and Using Props:
1. Syntax for Declaring Props:
In Vue 3, there are two primary ways to declare props: within single-file components and using the options API. When using single-file components, we declare props in the component's props option, specifying the desired prop names and their respective types. On the other hand, when using the options API, we declare props within the props property of the component object.
2. Passing Props from Parent to Child Component:
To pass props from a parent component to a child component, we employ the v-bind directive or the shorthand syntax. With v-bind, we can bind a prop to a dynamic value in the parent component, ensuring that any changes to the prop are reflected in the child component. Alternatively, we can use the shorthand syntax, which automatically binds the prop to the parent component's data property with the same name.
II. Common Issues with Props and How to Solve Them:
A. Undefined or Missing Props:
1. Handling Undefined or Missing Props Gracefully:
When dealing with props that may be undefined or missing, it is crucial to handle these scenarios gracefully. One approach is to provide default values or fallbacks for the props, ensuring that the child component can still function even if the prop is not passed from the parent. By setting default values, we can avoid unexpected errors and improve the overall user experience.
2. Validating Prop Types and Values:
Vue 3 provides a built-in mechanism for prop type validation, allowing us to catch potential issues early on. By specifying the expected type or an array of valid types for a prop, we can ensure that the correct data is passed from the parent component. Furthermore, we can also define custom validation functions to validate prop values based on specific requirements.
B. Prop Naming Collisions:
1. Avoiding Naming Conflicts Between Prop Names and Component Data/Methods:
To prevent naming conflicts between prop names and component data or methods, it is advisable to follow best practices for naming conventions. By using a consistent naming pattern, such as prefixing prop names with a specific identifier, we can minimize the risk of accidental collisions. Additionally, adopting a clear and descriptive naming scheme for props can enhance code readability and maintainability.
C. Modifying Props Directly (Anti-Pattern):
1. The Importance of Avoiding Direct Modification of Props:
In Vue, the data flow between parent and child components follows a one-way direction, emphasizing the concept of "one-way data flow." As a result, directly modifying props within child components is considered an anti-pattern. Instead, Vue encourages us to utilize computed properties or emit events to communicate changes back to the parent component. By adhering to this principle, we maintain a clear and predictable data flow, leading to more manageable and maintainable code.
III. Optimizing Performance with Props:
A. Avoiding Unnecessary Prop Updates:
1. Understanding the Reactivity System in Vue:
Vue 3's reactivity system plays a vital role in determining when and how props are updated. By understanding how reactivity works, we can optimize our applications by minimizing unnecessary prop updates. By default, Vue performs shallow comparisons to detect changes in props, but in certain scenarios, we can leverage the shouldUpdate() lifecycle hook to fine-tune the reactivity behavior.
2. Strategies for Minimizing Prop Updates:
To optimize performance, it is essential to minimize prop updates whenever possible. One strategy is to use object literals as props judiciously, as these can lead to reactivity issues. In cases where object literals are necessary, we can choose between shallow watch and deep watch, depending on the specific requirements of our application. Shallow watch allows us to monitor changes in the object's properties, while deep watch tracks changes within nested properties.
IV. Troubleshooting Tips and Tricks:
A. Debugging Props:
1. Using Vue Devtools to Inspect Props:
Vue Devtools is a powerful tool that aids in debugging and inspecting component props. By using Vue Devtools, we can gain valuable insights into the current state of our props, facilitating the identification and resolution of potential issues.
B. Console Logging for Prop Values:
1. Utilizing Console Logging Techniques for Prop Values:
During development and debugging, logging prop values can be immensely helpful. By incorporating console.log statements within our code, we can output prop values to the browser's console, allowing us to verify that the correct data is being passed from the parent component to the child component.
Conclusion:
In this blog post, we explored the importance of understanding and troubleshooting props in Vue 3. Props play a vital role in enabling efficient communication between parent and child components, fostering the development of reusable and modular code. By applying the tips and tricks shared in this article, developers can navigate common issues with props and optimize their applications for better performance. We encourage readers to experiment with props in their own projects and welcome any feedback, questions, or suggestions. Happy coding in Vue 3!
FREQUENTLY ASKED QUESTIONS
They allow you to communicate between components and make your application more modular.
Components play a crucial role in making applications more modular and facilitating communication between different parts. They serve as the building blocks of an application, allowing developers to break down complex functionalities into smaller, manageable pieces.By using components, you can compartmentalize different features or sections of your application, making it easier to understand, maintain, and update. Components can communicate with each other through APIs (Application Programming Interfaces), enabling seamless data exchange and interaction.
This modular approach not only enhances code reusability but also promotes scalability and flexibility. It allows developers to work on specific components independently, making development more efficient and collaborative.
In summary, components serve as the glue that connects different parts of your application, enabling effective communication and making your application more modular and adaptable to changes.
How do I pass props to a child component?
To pass props to a child component in React, you can follow these steps:
-
In your parent component, define the props that you want to pass to the child component. For example, let's say you have a parent component called "Parent" and you want to pass a prop called "message" to the child component.
-
Inside the parent component, when rendering the child component, use the JSX syntax to pass the props. You can do this by adding the prop name and its value as attributes to the child component. For example, if your child component is called "Child", you can pass the "message" prop like this:
<Child message="Hello from parent!" />
. -
In the child component, you can access the passed prop by using the props object. The props object contains all the props that were passed to the component. For example, in the "Child" component, you can access the "message" prop like this:
props.message
.
By following these steps, you can successfully pass props from a parent component to a child component in React. This allows you to share data and information between different components in your application.
What should I do if my child component is not receiving props?
If your child component is not receiving props, there are a few things you can try to troubleshoot the issue.First, double-check that you are correctly passing the props from the parent component to the child component. Make sure you are using the correct prop names and that you are passing the props in the correct format.
Next, verify that the child component is expecting to receive the props you are trying to pass. Check the child component's code to see if it has defined the props it expects to receive. If the child component is not explicitly looking for the props, it won't be able to access them.
If you have confirmed that you are passing the props correctly and the child component is set up to receive them, it's possible that there may be an issue with the component hierarchy or the way the components are nested. Make sure the parent component is rendering the child component correctly and that there are no missing or extra layers in the component structure.
If none of the above solutions work, it may be helpful to use debugging tools or console.log statements to inspect the props at various points in the component hierarchy. This can help you pinpoint where the issue is occurring and provide more information for troubleshooting.
Remember, it's important to have a clear understanding of how props are passed and received in React components. Taking the time to review the React documentation or seeking guidance from online resources can also be helpful in resolving any issues you may encounter.
How can I make props reactive in Vue 3?
To make props reactive in Vue 3, you can utilize the Composition API's ref
function. First, import the ref
function from the vue
package:
import { ref } from 'vue';
Next, declare a ref
variable for each prop you want to make reactive. For example, let's say we have a prop called message
:
props: {
message: {
type: String,
required: true
}
},
setup(props) {
const reactiveMessage = ref(props.message);
// ...
}
Now, you can use the reactiveMessage
variable just like any other reactive variable in Vue. Any changes made to props.message
will be automatically reflected in reactiveMessage
.
Remember to pass the reactiveMessage
variable to your template or component as needed.
Hope this helps! Let me know if you have any other questions.